- Joined
- Feb 25, 2016
- Messages
- 1,437
- Likes
- 2,887
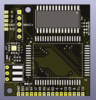
PCB: https://github.com/Gmanmodz/N64-Controller-Module
Joystick Converter MCU: https://github.com/Gmanmodz/N64-Joystick-Converter
For Joystick Calibration:
The joystick will not function properly until it has been calibrated.
Hold Z+R while powering on to enter calibration mode. Release Z+R. Swirl the joysticks a few times. Press Z+R again to store values.